Google Login Setup
Questo contenuto non è ancora disponibile nella tua lingua.
Introduction
In this guide, you will learn how to setup Google Login with Capgo Social Login. You will need the following in order to setup Google Login:
- A Google account
General setup
In this part, you will setup the login screen displayed by Google.
- Please go to console.cloud.google.com
- Click on the project selector
- If you don’t have a project already, please create a new project.
- Click on
New project
- Name your project and click
Create
- Ensure that you are on the right project
- Click on
- Start to configure the
OAuth consent screen
-
Click on the search bar
-
Search for
OAuth consent screen
and click on it -
Configure the consent screen
Click on
create
-
- Fill the information about your app
-
Let’s start with the
App Information
- Please type in your
App Name
- Enter the
user support email
-
You CAN add the app logo.
-
You SHOULD configure the
App domain
-
You HAVE TO provide the developer’s email
-
Click on
save and continue
- Please type in your
-
- Configure the scopes
-
Click on
add or remove scopes
-
Select the following scopes and click
update
-
Click
save and continue
-
- Add a test user
- Click on
add users
- Enter your Google email, click enter, and click
add
- Click
save and continue
- Click on
- Click
back to dashboard
- Submit your app for verification
Differences between online access and offline access
There are multiple ways to use Google Login with Capacitor. Here is a table that summarizes the differences between the two:
Online access | Offline access | |
---|---|---|
Requires a backend | ❌ | ✅ |
Long-lived access token | ❌ | ✅ |
Easy setup | ✅ | ❌ |
If you still do not know which one you should choose, please consider the following scenarios:
-
You want the user to login, immediately after you are going to issue him a custom JWT. Your app will NOT call Google APIs
In this case, choose online access.
-
Your app will call some Google APIs from the client, but never from the backend
In this case, choose online access
-
Your app will call some google APIs from the backend, but only when the user is actively using the app
In this case, choose online access
-
Your app will periodically check the user’s calendar, even when he is not actively using the app
In this case, choose offline access
An example backend for online access
In this part of the tutorial, I will show how to validate the user on your backend.
This example will be very simple and it will be based on the following technologies:
You can find the code for this example here
As you can see:
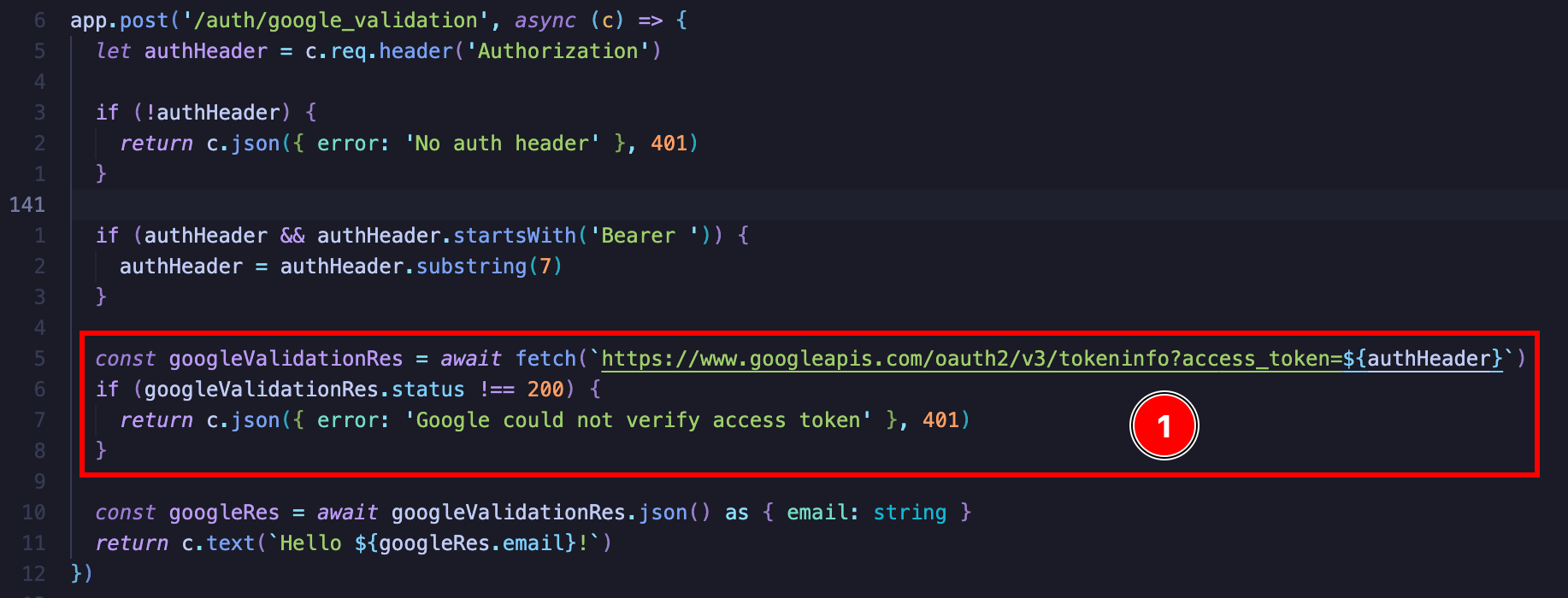
The idea is rather simple. You send a simple GET
request to https://www.googleapis.com/oauth2/v3/tokeninfo
and this returns you whether the token is valid or not and if it it is, it gives you the email of the user. It also gives you some other info about the user token

From there, you could issue the user with your own JWT or issue some sort of session cookie. The possibilities are endless, for the final auth implementation.
If you do want to call Google API’s, I would strongly recommend looking at Google’s OAuth 2.0 Playground. From there you can easily see what APIs you can call.
Using offline access with your own backend
In order to use online access you will need the following:
- An HTTP server
In this example, I will be using the following technologies to provide the offline access in my app:
-
LowDb (a simple database)
The code for this example can be found here
As for the client code, it looks like this:
import { Capacitor } from '@capacitor/core';import { GoogleLoginOfflineResponse, SocialLogin } from '@capgo/capacitor-social-login';import { usePopoutStore } from '@/popoutStore'; // <-- specific to my app
const baseURL = "[redacted]";
async function fullLogin() { await SocialLogin.initialize({ google: { webClientId: '[redacted]', iOSClientId: '[redacted]', iOSServerClientId: 'The same value as webClientId', mode: 'offline' // <-- important } }) const response = await SocialLogin.login({ provider: 'google', options: { forceRefreshToken: true // <-- important } })
if (response.provider === 'google') { const result = response.result as GoogleLoginOfflineResponse const res = await fetch(`${baseURL}/auth/google_offline`, { headers: { "Content-Type": "application/json" }, body: JSON.stringify({ serverAuthCode: result.serverAuthCode, platform: Capacitor.getPlatform() }), method: "POST" })
if (res.status !== 200) { popoutStore.popout("Full google login failed", "check console"); return }
const { jwt } = await res.json(); const userinfo = await fetch(`${baseURL}/auth/get_google_user`, { headers: { Authorization: `Bearer ${jwt}` } }) if (userinfo.status !== 200) { popoutStore.popout("Full google (userinfo) login failed", "check console"); return } popoutStore.popout("userinfo res", await userinfo.text()); }}